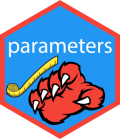
Simulated draws from model coefficients
Source:R/5_simulate_model.R
, R/methods_glmmTMB.R
simulate_model.Rd
Simulate draws from a statistical model to return a data frame of estimates.
Usage
simulate_model(model, iterations = 1000, ...)
# S3 method for class 'glmmTMB'
simulate_model(
model,
iterations = 1000,
component = c("all", "conditional", "zi", "zero_inflated", "dispersion"),
verbose = FALSE,
...
)
Arguments
- model
Statistical model (no Bayesian models).
- iterations
The number of draws to simulate/bootstrap.
- ...
Arguments passed to
insight::get_varcov()
, e.g. to allow simulated draws to be based on heteroscedasticity consistent variance covariance matrices.- component
Should all parameters, parameters for the conditional model, for the zero-inflation part of the model, or the dispersion model be returned? Applies to models with zero-inflation and/or dispersion component.
component
may be one of"conditional"
,"zi"
,"zero-inflated"
,"dispersion"
or"all"
(default). May be abbreviated.- verbose
Toggle warnings and messages.
Details
Technical Details
simulate_model()
is a computationally faster alternative
to bootstrap_model()
. Simulated draws for coefficients are based
on a multivariate normal distribution (MASS::mvrnorm()
) with mean
mu = coef(model)
and variance Sigma = vcov(model)
.
Models with Zero-Inflation Component
For models from packages glmmTMB, pscl, GLMMadaptive and
countreg, the component
argument can be used to specify
which parameters should be simulated. For all other models, parameters
from the conditional component (fixed effects) are simulated. This may
include smooth terms, but not random effects.
Examples
model <- lm(Sepal.Length ~ Species * Petal.Width + Petal.Length, data = iris)
head(simulate_model(model))
#> (Intercept) Speciesversicolor Speciesvirginica Petal.Width Petal.Length
#> 1 3.697081 -0.7427851 -1.916295 0.01678993 0.8651877
#> 2 3.561323 -0.8868297 -2.204739 0.89345532 0.8459629
#> 3 3.735007 -1.1030224 -1.507661 0.37943346 0.7891903
#> 4 3.370805 -1.2357496 -2.634898 0.66457033 1.0104691
#> 5 3.562752 -0.4832967 -2.958752 0.20377571 0.9696609
#> 6 3.535843 -1.4067392 -2.100093 0.40516218 0.9324729
#> Speciesversicolor:Petal.Width Speciesvirginica:Petal.Width
#> 1 -0.5041445 -0.03449445
#> 2 -1.1510572 -0.65681340
#> 3 -0.3754822 -0.35400411
#> 4 -0.9979501 -0.58865989
#> 5 -1.1129731 0.10677285
#> 6 -0.4764052 -0.37776701
# \donttest{
if (require("glmmTMB", quietly = TRUE)) {
model <- glmmTMB(
count ~ spp + mined + (1 | site),
ziformula = ~mined,
family = poisson(),
data = Salamanders
)
head(simulate_model(model))
head(simulate_model(model, component = "zero_inflated"))
}
#> (Intercept) minedno
#> 1 0.8453276 -1.856484
#> 2 0.2201298 -1.418678
#> 3 0.8480031 -2.148804
#> 4 0.4246136 -1.452492
#> 5 0.6706626 -1.845663
#> 6 0.7750347 -1.777401
# }